Using the Python interpreter
The Python shell
The Python interpreter provides you with an environment for experimentation and observation—the Python shell, where we work in interactive mode. It’s a great way to get your feet wet.
When working with the Python shell, you can enter expressions and Python will read them, evaluate them, and print the result. (There’s more you can do, but this is a start.)
There are several ways to run the Python shell: in a terminal (command prompt) by typing python
, python3
, or py
depending on the version(s) of Python installed on your machine. You can also run the Python shell through your chosen IDE (details will vary).
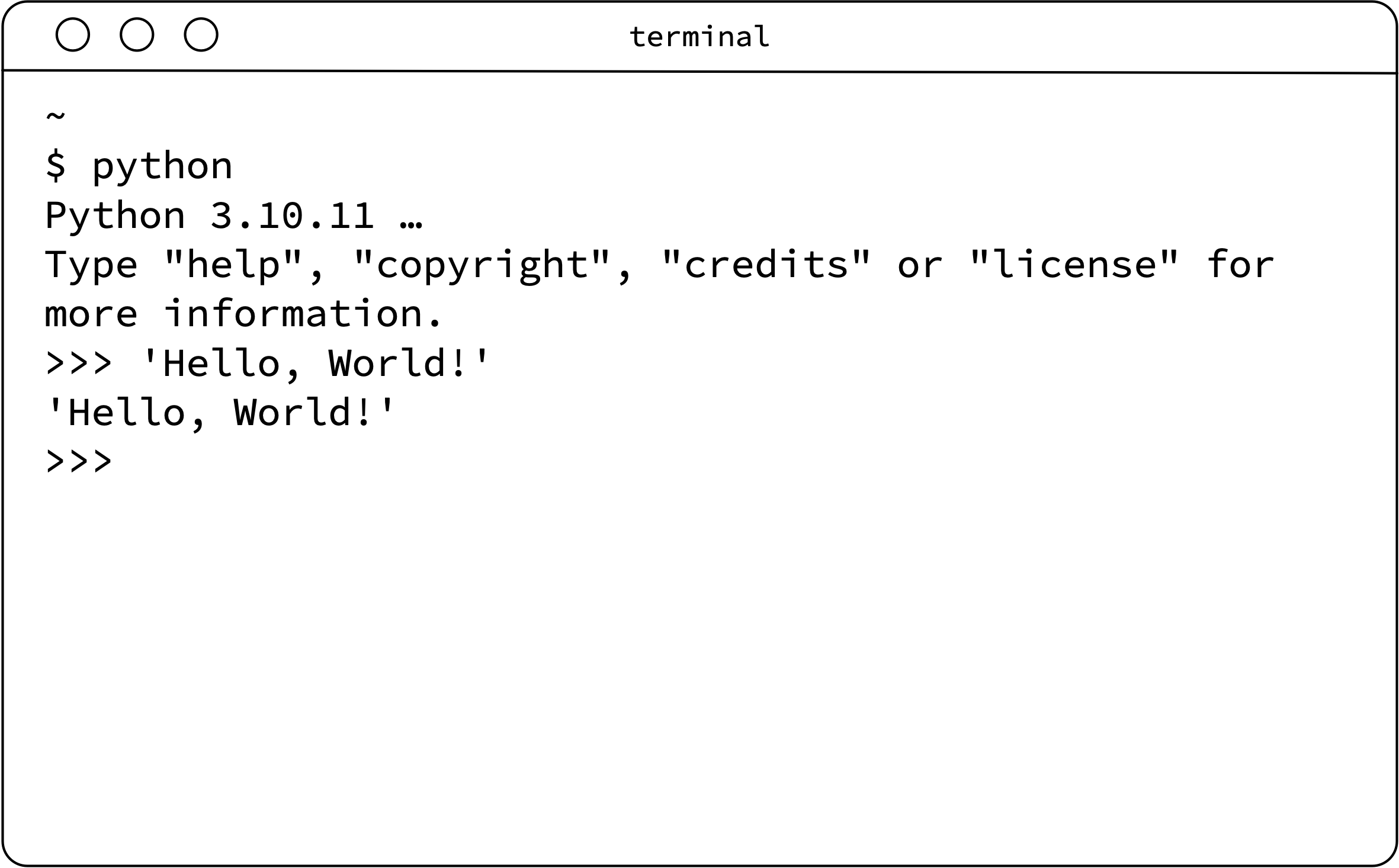
The first thing you’ll notice is this symbol: >>>
. This is the Python prompt (you don’t type this bit, this is Python telling you it’s ready for new input).
We’ll start with some simple examples (open the shell on your computer and follow along):
>>> 1
1
Here we’ve entered 1
. This is interpreted by Python as an integer, and Python responds by printing the evaluation of what you’ve just typed: 1
.
When we enter numbers like this, we call them “integer literals”—in the example above, what we entered was literally a 1
. Literals are special in that they evaluate to themselves.
Now let’s try a simple expression that’s not a mere literal:
>>> 1 + 2
3
Python understands arithmetic and when the operands are numbers (integers or floating-point) then +
works just like you’d expect. So here we have a simple expression—a syntactically valid sequence of symbols that evaluates to a value. What does this expression evaluate to? 3
of course!
We refer to the +
operator as a binary infix operator, since it takes two operands (hence, “binary”) and the operand is placed between the operands (hence, “infix”).
Here’s another familiar binary infix operator: -
. You already know what this does.
>>> 17 - 5
12
Yup. Just as you’d expect. The Python shell evaluates the expression 17 - 5
and returns the result: 12
.
REPL
This process—of entering an expression and having Python evaluate it and display the result—is called REPL which is an acronym for read-evaluate-print loop. Many languages have REPLs, and obviously, Python does too. REPLs were invented (back in the early 1960s) to provide an environment for exploratory programming. This is facilitated by allowing the programmer to see the result of each portion of code they enter. Accordingly, I encourage you to experiment with the Python shell. Do some tinkering and see the results. You can learn a lot by working this way.
Saving your work
Entering expressions into the Python shell does not save anything. In order to save your code, you’ll want to work outside the shell (we’ll see more on this soon).
Exiting the interpreter
If you’re using an IDE there’s no need to exit the shell. However, if you’re using a terminal, and you wish to return to your command prompt, you may exit the shell with exit()
.
>>> exit()
Copyright © 2023–2025 Clayton Cafiero
No generative AI was used in producing this material. This was written the old-fashioned way.